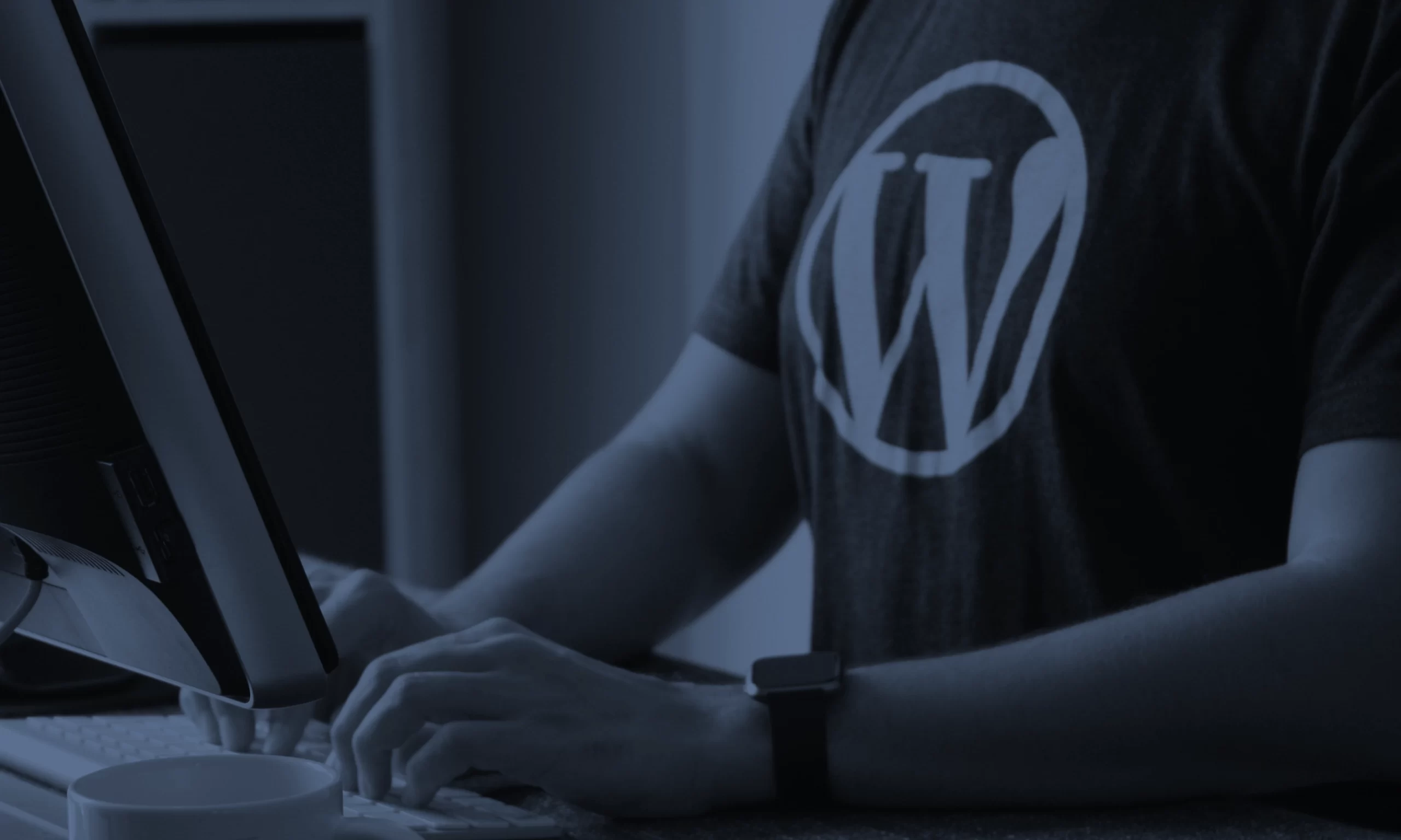
Adding a Password Input to WordPress Settings
WordPress Settings
In previous articles in the WordPress Settings series we covered adding a settings page with a few basic fields. Those articles cover the steps to build a settings form with three text inputs, a textarea input field, and a WP Editor field. This article builds on the previous articles by adding a Password field to our existing settings.
You may be asking why you need a Password field in your settings. I find the password field useful when adding fields for API Keys or other API authentication settings. So let’s dive in!
Adding the Defaults
In the previous article we covered how every WordPress setting should have a default value assigned and how to add the defaults to your code. Below is an extended version of that code that includes the new default settings for our Password input.
<?php
function ltdi_register_settings() {
/* Code for settings section goes here. */
if ( false == get_option( 'ltdi_theme_settings' ) ) {
$defaults = [
'setting_field_one' => '',
'setting_field_two' => '',
'setting_field_three'. => '', /* removed code */
'setting_textarea_field' => '',
'setting_wp_editor_field' => '',
'setting_password_field' => '', /* new code */
];
add_option( 'ltdi_theme_settings', $defaults );
}
}
add_action( 'admin_init', 'ltdi_register_settings' );
What is this code doing:
- We continue editing the “ltdi_register_settings” function from the previous article by adding a key/value pair for our setting_password_field.
- The default value should be an empty string.
- Like the previous article, if no settings are found, the get_options() function will return false.
- When a false value is returned from get_option() we use the function add_option(), passing our settings unique identifier and the array of defaults as the first and second parameters respectively. Doing so will save the default settings to the database.
Note: I have removed one of the text fields added in an earlier article and replaced it with the new default. Anything I’ve removed is indicated with comment “removed code”, and anthing I have added is indicated with the comment “new code”.
Adding the Password Input
As mentioned in the previous article’s section “Adding the Setting Input Fields”, each field is added with a combination of the built-in function “add_settings_field” and a callback function for the specific field type.
Add Settings Field
For the password input we start with the built-in function add_settings_field, using the arguments listed below.
$name
The first argument is the field $name. It is a required unique identifier for the setting. It should be a single string value usually formatted with underscores to combine multiple words. In this case it is “setting_password_field”.
$title
The second argument is the field $title. It should be a string value formatted for display to the WordPress user. For this tutorial we will use the string “New Password Setting”.
$callback
The third argument is the name of the $callback function that outputs the form input’s HTML. Because we are implementing a Password input we will be using the name “ltdi_get_password_input”.
$option_name
The fourth argument tells WordPress what settings group to add our setting to. For this tutorial we will use the string “ltdi_theme_settings” which is the name of our settings group.
$section_name
The fifth argument tells WordPress what section to associate our setting with. For this tutorial we will use the string “ltdi_theme_settings_section” which is the name of our settings section.
$args
For the last argument we use an array with two key/value pairs, one with the key “label_for”, and one with the key “class”. The “label_for” key is used by WordPress to create HTML label tags around the form label. The provided value is used as the “for” attribute in that tag. The “class” key is used by WordPress to add a custom CSS Class attribute to the wrapping <tr> HTML tags around the input.
<?php
function ltdi_register_settings() {
/* Code for settings section adding defaults goes here. */
/* Code that adds the text fields in the previous article goes here. */
add_settings_field(
'setting_password_field',
'New Password Setting',
'ltdi_get_password_input',
'ltdi_theme_settings',
'ltdi_theme_settings_section',
[
'label_for' => 'setting_password_field',
'class' => 'setting_password_field'
]
);
Adding the Password Callback
Although the field has been added to WordPress, before it can be used we need to create the callback function to output the password input’s HTML on the screen.
<?php
function ltdi_get_password_input($args) {
$settings = \get_option('ltdi_theme_settings');
$value = $settings[$args['label_for']] ?? '';
printf(
'<input type="password" id="%1$s" name="ltdi_theme_settings[%1$s]" value="%2$s" />',
$args['label_for'],
$value
);
}
What is this code doing:
- We start by declaring the function “ltdi_get_password_input”.
- We then use the get_option() function with the argument “ltdi_theme_settings” to retrieve all our settings.
- We check the existence of the field’s value using the Null Coalescing Operator, assigning its value or an empty string to the variable $value.
- We finallly use the printf() function to print the password HTML input tag, filling in the ID and name attributes with the “label_for” argument, and the value attributes with our previously assigned $value variable.
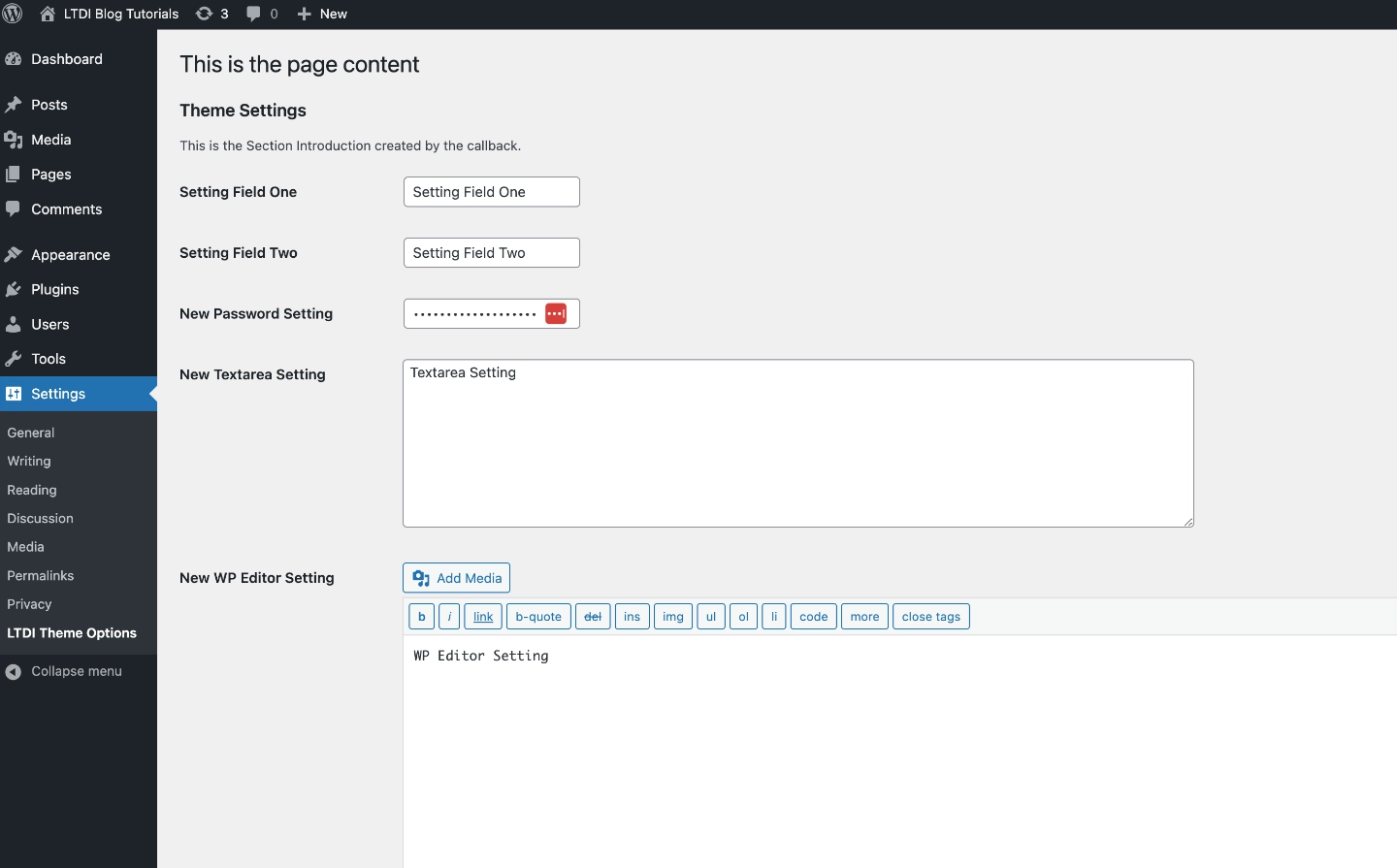
Putting It All Together
The final code is available for download on github. When this code is added to your WordPress theme’s function file or your plugin’s main file, you should have a functioning WordPress settings page with two text inputs, one textarea input, one WordPress Editor input, and our new password input.
Remember: something as simple as a typo can take down a website. For this reason I recommend plenty of backups. I also recommend using a development environment to test the code before pushing it live.
Receive Tutorials Direct to Your Inbox