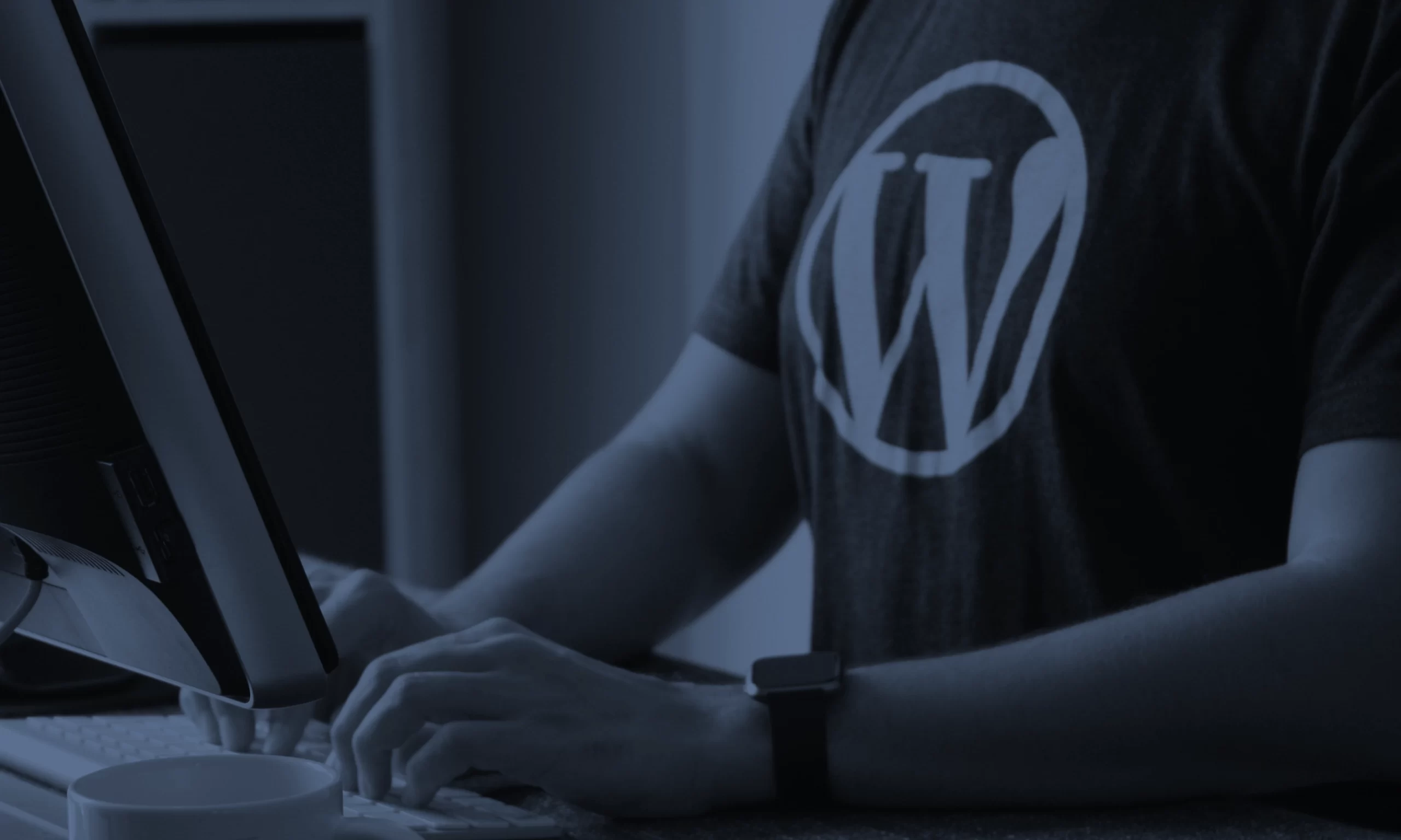
Adding a Textarea Input to WordPress Settings
WordPress Settings
In a previous article we covered adding WordPress settings without a plugin. That article demonstrated the required steps for building a settings form on a WordPress settings page including a simple text input repeated three times.
This article will build on the previous article by adding a textarea HTML input to our existing settings.
Adding the Defaults
In the previous article we covered how every WordPress setting should have a default value assigned and how to add the defaults to your code. Below is an extended version of that code that includes the default settings for our new Textarea input.
<?php
function ltdi_register_settings() {
/* Code for settings section goes here. */
if ( false == get_option( 'ltdi_theme_settings' ) ) {
$defaults = [
'setting_field_one' => '',
'setting_field_two' => '',
'setting_field_three'. => '',
'setting_textarea_field' => '' /* New Default */
];
add_option( 'ltdi_theme_settings', $defaults );
}
}
add_action( 'admin_init', 'ltdi_register_settings' );
What is this code doing:
- We continue editing the “ltdi_register_settings” function from the previous article by adding a key/value pair for our “setting_textarea_field”.
- The default value should be an empty string.
- Like the previous article, we check for our settings using the get_option() function. If no settings are found, the get_option() function will return false.
- When the conditional evaluates to false, we use the function add_option(), passing our settings unique identifier and the array of defaults as the first and second parameters respectively. Doing so saves the default settings to the database.
Adding the Textarea Input
As mentioned in the previous article’s section “Adding the Setting Input Fields”, each field is added with a combination of the built-in function “add_settings_field” and a callback function for the specific field type.
Add Settings Field
For the textarea input we start with the built-in function add_settings_field, using the arguments listed below.
$name
The first argument is the $name. It is a required unique identifier for the setting. It should be a single string value, usually formatted with underscores to combine multiple words. In this case it is “setting_textarea_field”.
$title
The second argument is the $title. It should be a string value formatted for display to the WordPress user. For this tutorial we will use the string “New Textarea Setting”.
$callback
The third argument is the name of the $callback function that outputs the input’s HTML. Because we are implementing a Textarea input we will be using the name “ltdi_get_textarea_input”.
$option_name
The fourth argument tells WordPress what group of settings to add the setting to. For this tutorial we will use the string “ltdi_theme_settings” which is the name of our settings group.
$section_name
The fifth argument tells WordPress what section to associate our setting with. For this tutorial we will use the string “ltdi_theme_settings_section” which is the name of our settings section.
$args
For the last argument we use an array with two key/value pairs, one with the key “label_for”, and one with the key “class”.
The “label_for” key is used by WordPress to create HTML label tags for our field. The provided value is used as the “for” attribute in that tag.
The “class” key is used by WordPress to add a custom CSS Class attribute to the <tr> HTML tag that wraps the input.
<?php
function ltdi_register_settings() {
/* Code for settings section adding defaults goes here. */
/* Code that adds the text fields in the previous article goes here. */
add_settings_field(
'setting_textarea_field',
'New Textarea Setting',
'ltdi_get_textarea_input',
'ltdi_theme_settings',
'ltdi_theme_settings_section',
[
'label_for' => 'setting_textarea_field',
'class' => 'setting_textarea_field'
]
);
Adding the Textarea Callback
Although the field has been added to WordPress, before it can be used we need to create the callback function to output the textarea HTML on the screen.
<?php
function ltdi_get_textarea_input($args) {
$settings = \get_option('ltdi_theme_settings');
$value = $settings[$args['label_for']] ?? '';
printf(
'<textarea id="%1$s" name="ltdi_theme_settings[%1$s]" rows="8" cols="100">%2$s</textarea>',
$args['label_for'],
$value
);
}
What is this code doing:
- We start by declaring the function “ltdi_get_textarea_input”.
- We then use the get_option() function with the argument “ltdi_theme_settings” to retrieve our settings.
- We then use a ternary and the “label_for” key to see if the specific setting value exists.
- If a value exists, it is assigned to the $value variable, if not an empty string is assigned.
- We finallly use the printf() function to print the TextArea HTML tag, filling in the ID and name attributes with the stored value of the “label_for” key, and the value from our previously assigned $value variable.
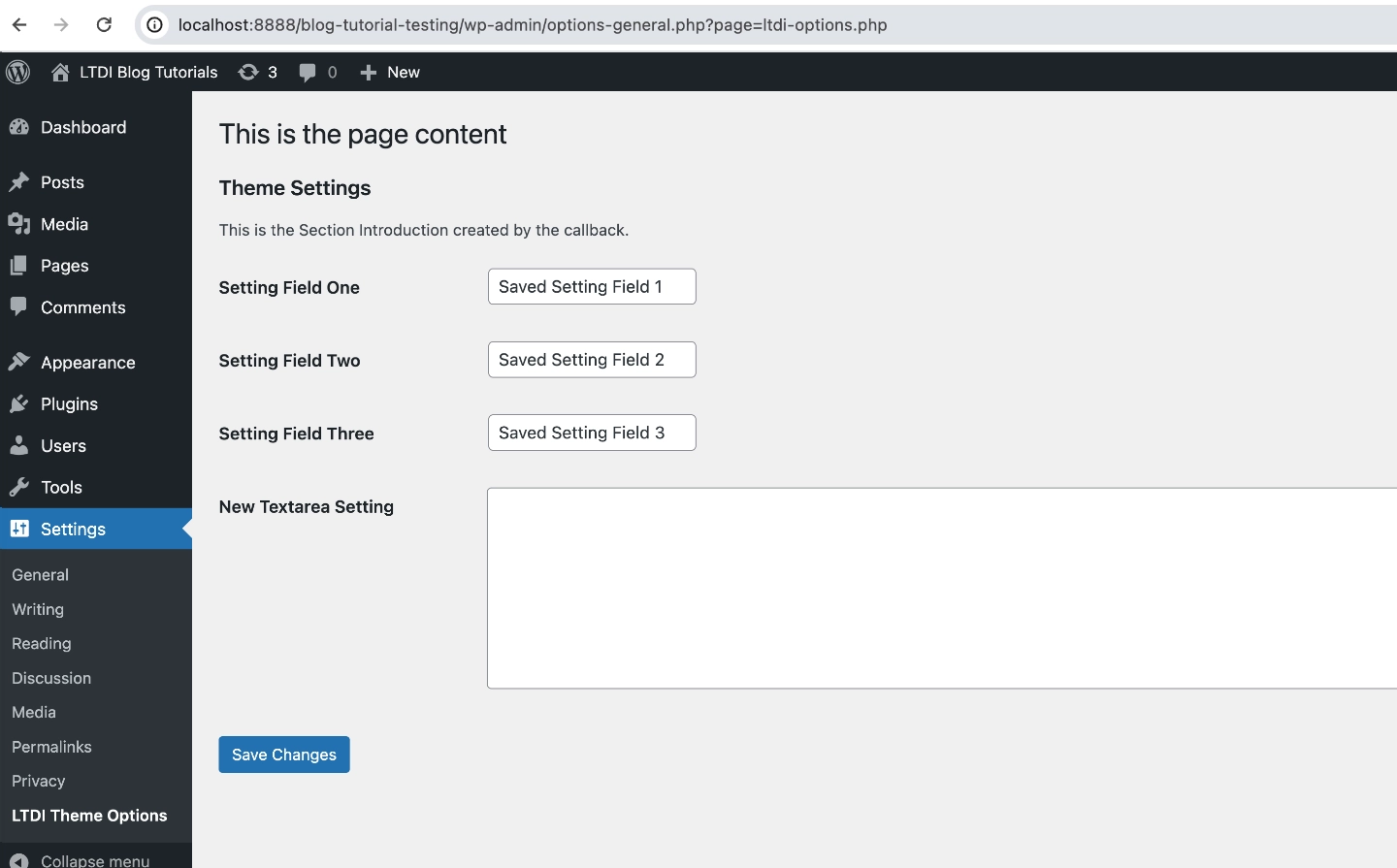
Putting It All Together
Here is the final code including code from the previous article. What is added in this article is indicated with comment “new code”. When this code is added to your WordPress theme’s function file or your plugin’s main file, you should have a functioning WordPress settings page with three text fields and one textarea field.
Remember: something as simple as a typo can take down a website. For this reason I recommend plenty of backups. I also recommend using a development environment to test the code before pushing it live.
<?php
function ltdi_register_settings() {
add_settings_section(
'ltdi_theme_settings_section',
__('Theme Settings', 'ltdi'),
'ltdi_section_introduction'
'ltdi_theme_settings'
);
if ( false == get_option( 'ltdi_theme_settings' ) ) {
$defaults = [
'setting_field_one' => '',
'setting_field_two' => '',
'setting_field_three' => '',
'setting_textarea_field' => '' /* New Code */
];
add_option( 'ltdi_theme_settings', $defaults );
}
add_settings_field(
'setting_field_one',
__('Setting Field One', 'ltdi'),
'ltdi_get_text_input',
'ltdi_theme_settings',
'ltdi_theme_settings_section',
[
'label_for' => 'setting_field_one',
'class' => 'setting_field_one'
]
);
add_settings_field(
'setting_field_two',
__('Setting Field Two', 'ltdi'),
'ltdi_get_text_input',
'ltdi_theme_settings',
'ltdi_theme_settings_section',
[
'label_for' => 'setting_field_two',
'class' => 'setting_field_two'
]
);
add_settings_field(
'setting_field_three',
__('Setting Field Three', 'ltdi'),
'ltdi_get_text_input',
'ltdi_theme_settings',
'ltdi_theme_settings_section',
[
'label_for' => 'setting_field_three',
'class' => 'setting_field_three'
]
);
/* New Code */
add_settings_field(
'setting_textarea_field',
__('New Textarea Setting', 'ltdi'),
'ltdi_get_textarea_input',
'ltdi_theme_settings',
'ltdi_theme_settings_section',
[
'label_for' => 'setting_textarea_field',
'class' => 'setting_textarea_field'
]
);
register_setting(
'ltdi_theme_settings',
'ltdi_theme_settings',
'ltdi_setting_sanitization'
);
}
add_action( 'admin_init', 'ltdi_register_settings' );
function ltdi_section_introduction(){
echo 'This is the Section Introduction'
}
function ltdi_get_text_input($args){
$settings = \get_option('ltdi_theme_settings');
$value = !empty($settings[$args['label_for']]) ?: '';
printf(
'<input type="text" id="%1$s" name="ltdi_theme_settings[%1$s]" value="%2$s" />',
$args['label_for'],
$value
);
};
/* New Code */
function ltdi_get_textarea_input($args) {
$settings = \get_option('ltdi_theme_settings');
$value = !empty($settings[$args['label_for']]) ?: '';
printf(
'<textarea id="%1$s" name="ltdi_theme_settings[%1$s]" rows="8" cols="100">%2$s</textarea>',
$args['label_for'],
$value
);
}
function ltdi_setting_sanitization($input) {
$output = [];
foreach( $input as $key => $value ) {
if (isset( $input[$key])) {
$output[$key] = strip_tags( stripslashes( $input[ $key ] ) );
}
}
return apply_filters( 'ltdi_setting_validation', $output, $input );
}
function ltdi_settings_page_callback() {
echo '<div class="wrap">';
echo __('<h1>This is the page content</h1>', 'ltdi');
echo '<form method="post" action="options.php">';
settings_fields('ltdi_theme_settings');
do_settings_sections('ltdi_theme_settings');
submit_button();
echo '</form>';
echo '</div>';
}
Get Content Direct to Your Inbox